How to send interrupt from Arduino and receive to C#
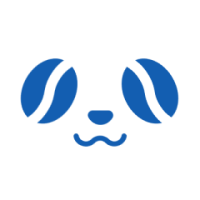
I want to use the Arduino (Lattepanda Leonardo) internal timer to generate an interrupt for every 20 milliseconds and accordingly want to receive this interrupt as a message in C# WPF. Using TimerOne.h and Firmata.h header files, I can able to create an interrupt for every 20 milliseconds and send a message "InterruptOccurred" (using Firmata.sendString("InterruptOccurred"); ) from Arduino. But using Lattepanda.Firmata package in WPF, C# I cannot able to receive the interrupt message in WPF. The code for Arduino and C# is attached herewith. Eagerly waiting for your valuable suggestions.
//Arduino code
#include <Firmata.h>
#include <TimerOne.h>
const long interval = 20; // Time interval in milliseconds
const int numberOfInterrupts = 20; // Number of interrupts to execute
volatile int interruptCounter = 0;
void setup() {
Firmata.setFirmwareVersion(FIRMATA_FIRMWARE_MAJOR_VERSION, FIRMATA_FIRMWARE_MINOR_VERSION);
Firmata.begin(57600);
Timer1.initialize(interval * 1000); // Timer interval is in microseconds
Timer1.attachInterrupt(timerISR); // Attach the ISR function
}
void loop() {
if (interruptCounter >= numberOfInterrupts) {
Timer1.stop();
Firmata.sendString("Timer stopped");
while (1) {} // Enter an infinite loop (halt the program)
}
}
void timerISR() {
// This function will be called every 20 milliseconds
Firmata.sendString("CustomEvent");
interruptCounter++;
}
// C# code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Threading;
using LattePanda.Firmata;
using System.IO.Ports;
namespace ArdIntrup
{
public partial class MainWindow : Window
{
private SerialPort serialPort;
public MainWindow()
{
InitializeComponent();
}
private void ConnectToArduino_Click(object sender, RoutedEventArgs e)
{
serialPort = new SerialPort("COM3", 57600);
serialPort.DataReceived += SerialPort_DataReceived;
try
{
serialPort.Open();
MessageBox.Show("Connected to Arduino");
}
catch (Exception ex)
{
MessageBox.Show("Error connecting to Arduino: " + ex.Message);
}
}
private void SerialPort_DataReceived(object sender, SerialDataReceivedEventArgs e)
{
string receivedData = serialPort.ReadLine();
Dispatcher.Invoke(() => {
// Update the TextBox with the received message
MessageTextBox.Text += receivedData + Environment.NewLine;
});
}
private void InitializeSerialPort()
{
serialPort = new SerialPort("COM3", 57600);
serialPort.DataReceived += SerialPort_DataReceived;
serialPort.Open();
}
// Close the serial port when closing the application
private void Window_Closing(object sender, System.ComponentModel.CancelEventArgs e)
{
if (serialPort != null && serialPort.IsOpen)
{
serialPort.Close();
}
}
}
}